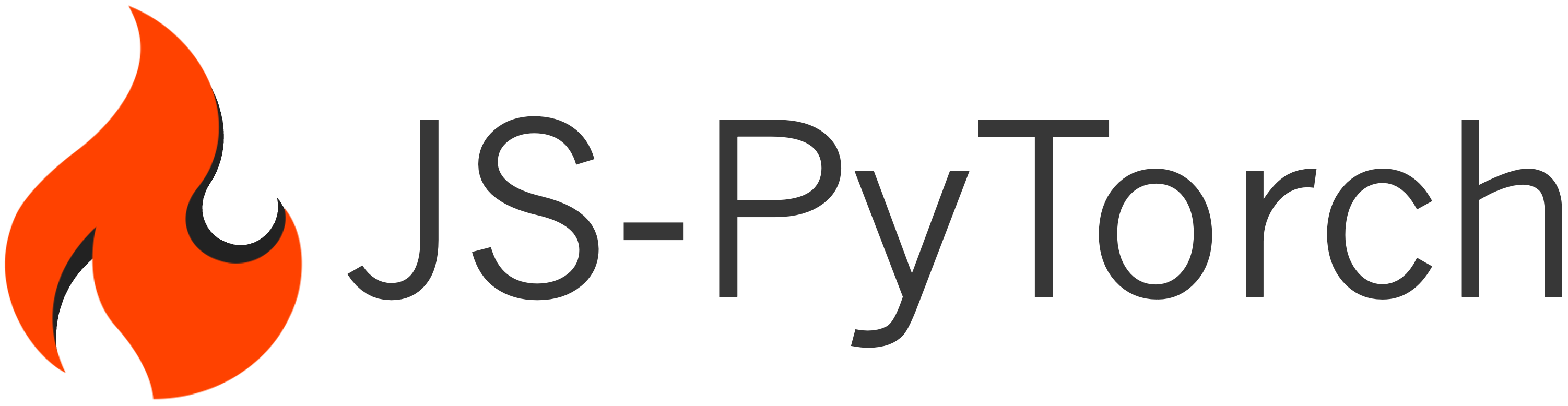
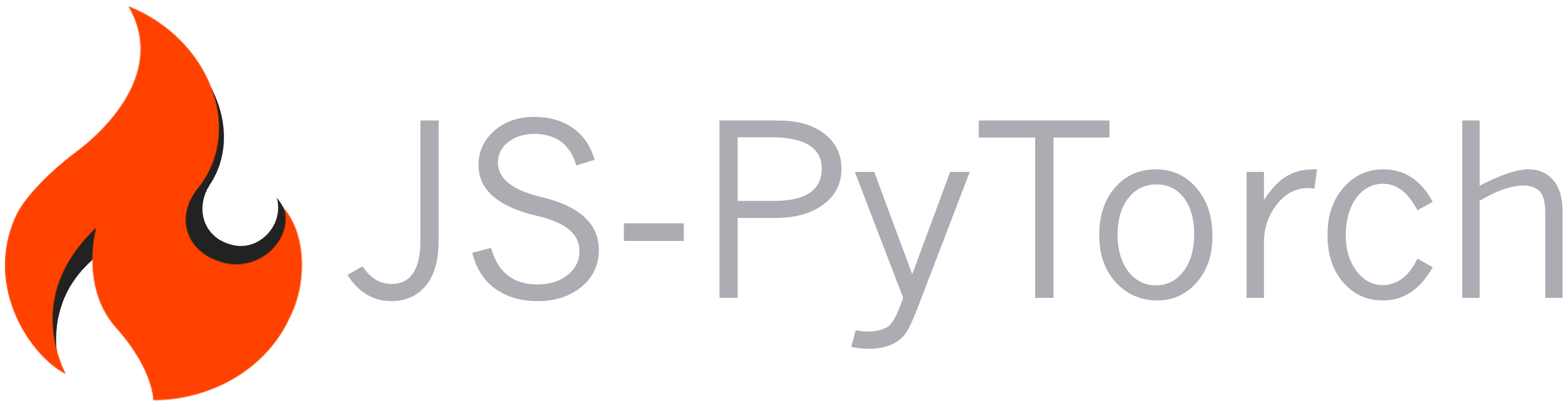
Deep Learning in JavaScript
About
- JS-PyTorch is a Deep Learning JavaScript library, which uses the seamless PyTorch syntax.
- This means that you can use this library to train, test and deploy Neural Networks, with node.js or on a web browser.
- For access to the source code, visit the GitHub repo.
Installation
This is a node package, and can be installed with npm (Node Package Manager). It has full support of node 20.15.1, which is the latest LTS (Long-Term Support) node version, and more recent versions.
To run JS-PyTorch in the browser, paste the following tag in the head of your HTML file. You can also get the latest tag from the cdnjs website:
<script src="https://cdnjs.cloudflare.com/ajax/libs/js-pytorch/0.7.2/js-pytorch-browser.js"
integrity="sha512-l22t7GnqXvHBMCBvPUBdFO2TEYxnb1ziCGcDQcpTB2un16IPA4FE5SIZ8bUR+RwoDZGikQkWisO+fhnakXt9rg=="
crossorigin="anonymous"
referrerpolicy="no-referrer"></script>
After that, you can use JS-PyTorch freely in any script tag in your HTML file.
<head>
<title>My Project</title>
<!-- New tag goes here -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/js-pytorch/0.7.2/js-pytorch-browser.js"
integrity="sha512-l22t7GnqXvHBMCBvPUBdFO2TEYxnb1ziCGcDQcpTB2un16IPA4FE5SIZ8bUR+RwoDZGikQkWisO+fhnakXt9rg=="
crossorigin="anonymous"
referrerpolicy="no-referrer">
</script>
<!---->
</head>
<body>
<script>
<!-- Write JS-Pytorch code here, no need to install or require -->
let x = torch.randn([10,5])
let linear = new torch.nn.Linear(5,1,'gpu',true)
let z = linear.forward(x)
console.log(z.data)
</script>
</body>
MacOS
- First, install node with the command line, as described on the node website:
# installs nvm (Node Version Manager)
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.7/install.sh | bash
# download and install Node.js (you may need to restart the terminal)
nvm install 20
# verifies the right Node.js version is in the environment
node -v # should print `v20.15.1`
# verifies the right npm version is in the environment
npm -v # should print `10.7.0`
- Now, use npm to install Js-PyTorch locally:
# installs js-pytorch
npm install js-pytorch
# if needed, install older version of js-pytorch
nvm install js-pytorch@0.1.0
- Finally, require the package in your javascript file:
const { torch } = require("js-pytorch");
const nn = torch.nn;
const optim = torch.optim;
Linux
- First, install node with the command line, as described on the node website:
# installs nvm (Node Version Manager)
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.7/install.sh | bash
# download and install Node.js (you may need to restart the terminal)
nvm install 20
# verifies the right Node.js version is in the environment
node -v # should print `v20.15.1`
# verifies the right npm version is in the environment
npm -v # should print `10.7.0`
- Now, use npm to install Js-PyTorch locally:
# installs js-pytorch
npm install js-pytorch
# if needed, install older version of js-pytorch
nvm install js-pytorch@0.1.0
- Finally, require the package in your javascript file:
const { torch } = require("js-pytorch");
const nn = torch.nn;
const optim = torch.optim;
Windows
-
First, download node from the prebuilt installer on the node website:
-
Now, use npm to install Js-PyTorch locally:
# installs js-pytorch
npm install js-pytorch
# if needed, install older version of js-pytorch
nvm install js-pytorch@0.1.0
Note:If this throws an error, you might need to install the latest version of Visual Studio, including the "Desktop development with C++" workload.
- Finally, require the package in your javascript file:
const { torch } = require("js-pytorch");
const nn = torch.nn;
const optim = torch.optim;
Contributing
- If you have detected a bug on the library, please file a Bug Report using a GitHub issue, and feel free to reach out to me on my LinkedIn or email.
- If you would like to see a new feature in Js-PyTorch, file a New Feature issue.
- Finally, if you would like to contribute, create a merge request to the
develop
branch. I will try to answer as soon as possible. All help is really appreciated!